ViewModel in MVC is a class that contains the fields which are represented in the strongly-typed view.ViewModel in the MVC design pattern is very similar to a model. ViewModel is that we use a ViewModel only in rendering views.
In this article, I am going to discuss the ideas about ViewModel in MVC with examples. Please read our previous articles before moving on to this article where we discussed the ViewData in MVC. We are going to discuss the following contents which are related to ViewModel in MVC.
ViewModel
- ViewModel allows to transfer the custom model/complex data from controller to view.
- You must be create a strongly typed View which is using the data model List.
- ViewModel properties are represented as Label, Display etc in the View.
- ViewModel can have validation rules using data annotation.
- ViewModel Can have multiple objects from different models or data source.
- It is easy to rendering and maintenance.
There is no such difference between ViewModel and Model. Only ViewModel is used for transfer the data between Controller and View.
Let’s see the below example for creating/uses of ViewModel in MVC application.
The below EmployeeModel class file must be placed under the Models folder in ASP.NET MVC application.
ViewModel in ASP.NET MVC with Simple Model
Create a ViewModel under the model folder.
EmployeeModel.cs
public class EmployeeModel
{
public int Id { set; get; }
public string Name { set;get; }
}
Now we will create the respective controller in our project.
EmployeeController.cs
public class EmployeeController : Controller
{
public ActionResult SimpleModel ( )
{
EmployeeModel emp = new EmployeeModel() { Id = 1, Name = "King" };
return View(emp);
}
}
This is our final step, we will create a view for displaying the data to the end user.
SimpleModel.cshtml
@model MVCIntro.Models.EmployeeModel
@{
ViewBag.Title = "SimpleModel";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Simple Model</h2>
<hr />
@Html.DisplayNameFor(model => model.Id)
@Html.DisplayFor(model > model.Id)
@Html.DisplayNameFor(model => model.Name)
@Html.DisplayFor(model => model.Name)
Now we need to run our application and will get the output on the browser.
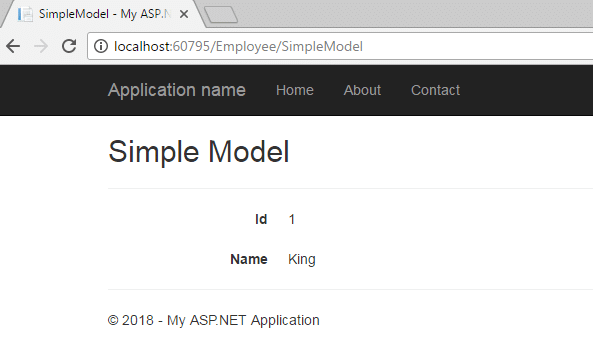
ViewModel in MVC with Complex Model
Create a ViewModel under the model folder.
EmployeeModel.cs
public class EmployeeModel
{
public int Id { set; get; }
public string Name { set;get; }
}
Now we will create the respective controller in our project.
EmployeeController.cs
public class EmployeeController : Controller
{
public ActionResult SimpleModel ( )
{
EmployeeModel emp = new EmployeeModel() { Id = 1, Name = "King" };
return View(emp);
}
public ActionResult ListModel ( )
{
List empList= new List()
{
new EmployeeModel{Id=1, Name="King"},
new EmployeeModel{Id=2, Name="Neil"},
new EmployeeModel{Id=3, Name="Rio"}
};
return View(empList);
}
}
This is our final step, we will create a view for displaying the data to the end-user.
ListModel.cshtml
@model IEnumerable mvcintro.models.employeemodel;
@{
ViewBag.Title = "ListModel";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>List Model</h2>
<table class="table">
<tbody>
<tr>
<th>@Html.DisplayNameFor(model => model.Id) </th>
<th>@Html.DisplayNameFor(model => model.Name) </th>
</tr>
<tr>
@foreach (var item in Model) {
<td>@Html.DisplayFor(modelItem => item.Id) </td>
<td>@Html.DisplayFor(modelItem => item.Name) </td>
}
</tr>
</tbody>
</table>
Now we need to run our application and will get the output on the browser.
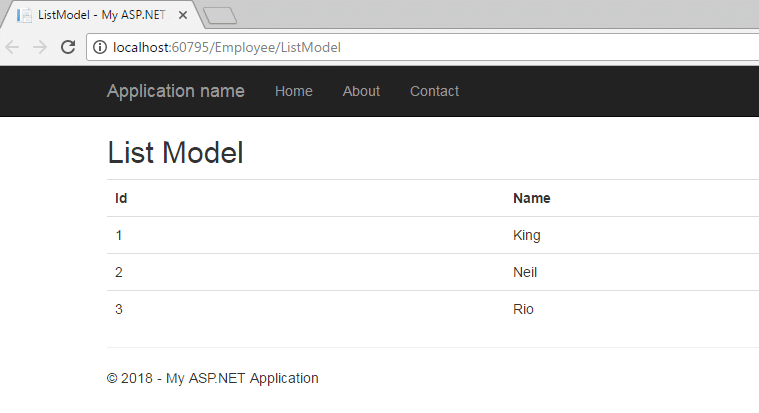
Warm UP – ViewModel in MVC
- ViewModel allows to transfer the custom model/complex data from controller to view.
- You must be create a strongly typed View which is using the data model List.
- ViewModel properties are represented as Label, Display etc in the View.
- ViewModel can have validation rules using data annotation.
- ViewModel Can have multiple objects from different models or data source.
- It is easy to rendering and maintenance.