The ViewBag in MVC is used to transfer temporary data from the controller to the view. ViewBag is a dynamic property and that enables you to share values dynamically between the controller and view within ASP.NET MVC applications.
In this article, I am going to discuss the ideas about ViewBag in MVC with Example. Please read our previous articles before moving on to this article where we discussed the different ways to pass data from Controller to View in MVC. we are going to discuss the following contents which are related to ViewBag in MVC.
ViewBag in MVC
- ViewBag can be used for transfer the data from controller to view and even view to view.
- ViewBag is a dynamic feature property of controllerbase class which was introduced in C# 4.0.
- It not requires typecasting for the complex object to read the data.
- It not require the null check
- It’s value becomes null when page is redirected.
- It’s live only during the current request.
- It is slower than ViewData.
- ViewBag is introduced in MVC 3.0 and available in MVC 3.0 and above
public dynamic ViewBag { get; }
This property defines under ControllerBase class.
Limitations of ViewBag
- It is not good pratice for bigger sets of data or complicated object because It is dynamic nature.
- Dynamic properties are not checked during the compilation time. So You may not be find the exact issue easily.
Let’s see the below example for creating/uses of ViewData in MVC application.
EmployeeController.cs
public class EmployeeController : Controller
{
public ActionResult Index ( )
{
ViewBag.Name = "www.infosyntax.com";
return View();
}
}
Index.cshtml
@{
ViewBag.Title = "Index";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Index</h2>
<h2>Welcome to @ViewBag.Name</h2>
Let’s the output on the above code.
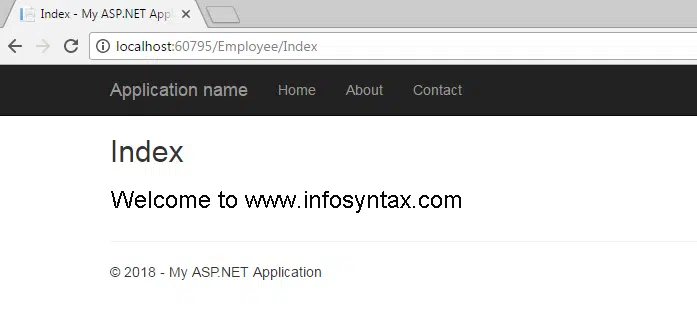
Bind Complex data into Viewbag in MVC
We can also use the complex data for assign to viewbag. Let’s see the below example.
EmployeeController.cs
public class EmployeeController : Controller
{
public ActionResult Index ( )
{
ViewBag.Name = "www.infosyntax.com";
return View();
}
public ActionResult ComplexIndex ( )
{
List Employee = new List();
Employee.Add("King");
Employee.Add("Lipsh");
Employee.Add("Rio");
ViewBag.Employee = Employee;
return View();
}
}
ComplexIndex.cshtml
@{
ViewBag.Title = "ComplexIndex";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>
Complex Index</h2>
<ul>
@foreach (var employee in ViewBag.Employee)
{
<li>@employee</li>
}
</ul>
Output-

Bind Custom Model into Viewbag in MVC
We can also bind custom models into viewbag. Let’s see the below example.
EmployeeController.cs
public class EmployeeController : Controller
{
public ActionResult Index ( )
{
ViewBag.Name = "www.infosyntax.com";
return View();
}
public ActionResult ComplexIndex ( )
{
List Employee = new List();
Employee.Add("King");
Employee.Add("Lipsh");
Employee.Add("Rio");
ViewBag.Employee = Employee;
return View();
}
public ActionResult customModelIndex ( )
{
ViewBag.Employee = Emp_List();
return View();
}
[NonAction]
private List Emp_List( )
{
List emps = new List()
{
new EmployeeModel{Id=1, Name="King"},
new EmployeeModel{Id=2, Name="Neil"},
new EmployeeModel{Id=3, Name="Rio"}
};
return emps;
}
}
customModelIndex.cshtml
@using MVCIntro.Models
@{
ViewBag.Title = "customModelIndex";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Custom Model Index</h2>
<ul>
@foreach (var emp in ViewBag.Employee)
{
<li> @emp.Name </li>
}
</ul>
Output-
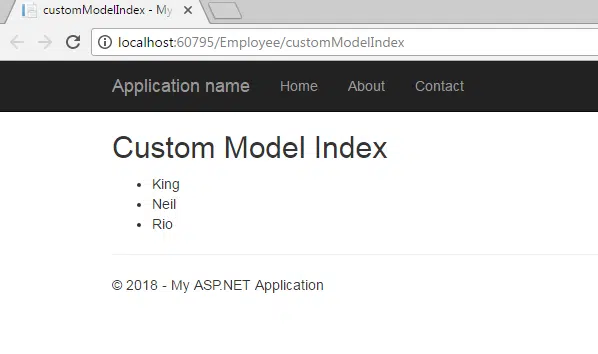
Warm UP – ViewBag in MVC
- ViewBag is a dynamic feature property of controllerbase class which was introduced in C# 4.0.
- It not requires typecasting for the complex object to read the data.
- It not require the null check
- It’s live only during the current request.
- It is slower than ViewData.
- Dynamic properties are not checked during the compilation time. So You may not be find the exact issue easily.