In this article, I am going to discuss the ideas about Advanced Routing in MVC with examples. Please read our previous articles before moving on to this article where we discussed Routing in ASP.NET MVC. As part of this article, we are going to discuss the following contents which are related to Advanced Routing in MVC.
Contents
Multiple Routes:
Route customization is an advanced topic. In this session, we will look at customize the multiple routing in the MVC application. You can configure the multiple routes using the Map Route extension method in RouteConfig.cs. This method needs at least two parameters such as route name and URL pattern and URL parameter is optional. If you need to pass some value to action using parameters then the parameter is mandatory.
While requested URLs come to the application. It is start compared to route patterns in order the pattern sequence in the route dictionary or order of added the route in RouteConfig.cs file. If request URLs are matched with the first URL pattern of the route dictionary, then it will call the specified controller and action. In case if URL is not matched with a pattern of the route dictionary, then it will call the default map route method and executed the default controller and action. Similarly, you can able to your own route map in routeconfig.cs file. Advanced Routing in MVC
Example -1
Route Configuring: – routeconfig.cs
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "EmployeeDetails",
url: "EmployeeDetails/{id}",
defaults: new { controller = "Employee", action = "Details", id = UrlParameter.Optional }
);
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional }
);
}
Attribute Routes:
ASP.NET MVC5 and WEB API 2 supports a new type of routing, called attribute routing. In this routing, attributes are used to define routes. Attribute routing provides you more control over the URIs by defining routes directly on actions and controllers in your ASP.NET MVC application and WEB API.
Enabling Attribute Routing
To enable Attribute Routing, we need to call the MapMvcAttributeRoutes method of the route collection class during configuration. Advanced Routing in MVC
- You must have enable attribute route in routeconfig.cs file.
- It must be enable before the default route.
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapMvcAttributeRoutes();
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Employee", action = "Index", id = UrlParameter.Optional }
);
}
Defining Attribute Routing in ASP.NET MVC
- Controller level attribute routing
- Action level attribute routing Advanced Routing in MVC
Controller level attribute routing
You can define routes at the controller level which apply to all actions within the controller unless a specific route is added to an action.
using System.Collections.Generic;
using System.Linq;
using System.Web.Mvc;
using ModelMVC.Models;
namespace ModelMVC.Controllers
{
[RoutePrefix("Employee")] //Route Prefix Employee
[Route("{action=index}")] //default action
public class EmployeeController : Controller
{
public ActionResult Index()
{
var empDetails = from emp in GetEmpolyeeDetails()
select emp;
return View(empDetails);
}
[NonAction]
public List GetEmpolyeeDetails()
{
List employeeList = new List()
{
new EmployeeModel {ID=1, Name="King", Location="London" },
new EmployeeModel {ID=2, Name="Lipsh", Location="Bangalore" },
new EmployeeModel {ID=3, Name="Rio", Location="Sydney" }
};
return employeeList;
}
//Route: /Employee/About
public ActionResult About()
{
ViewBag.Message = "Your application description page.";
return View();
}
}
}
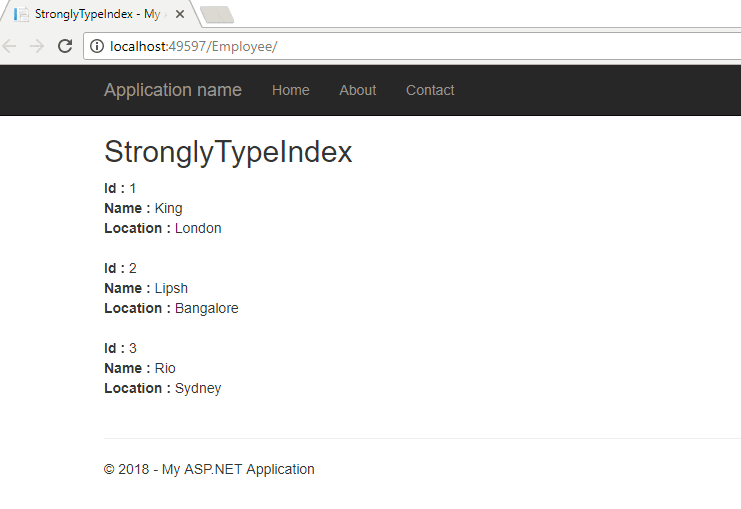
Action level attribute routing
You can define routes at the action level which apply to a specific action within the controller.
[Route("EmployeeDetails/{id}")]
public ActionResult EmployeeDetailsbyID(int id)
{
}
Pass Multiple Parameter to MVC Action:
Now we will discuss multiple parameter pass to action. you can define the rules in routeconfig.cs file and also attribute route.
For example, the following route applies a multiple parameter pass to action.
routeconfig.cs– Advanced Routing in MVC
public static void RegisterRoutes(RouteCollection routes)
{
routes.MapRoute(
"EmployeeByJoinDate",
"Employee/Join/{year}/{month}",
new { controller = "Employee", action = "EmployeeByJoinDate"});
}
Attribute Routes with Multiple Parameters:
In this case, you can also apply the rules in the controller on the attribute level.
[Route("Employee/Join/{year}/{month}")]
public ActionResult EmployeeByJoinDate(int year, int month)
{
}
Above both examples will work as same. Advanced Routing in MVC
Route Constraints:
It will restrict the value of parameters by configuring route constraints and attribute route constraints. The Route Constraint in ASP.NET MVC Routing allows us to apply a regular expression to a URL segment to restrict whether the route will match the request
Normal Route : Advanced Routing in MVC
public static void RegisterRoutes(RouteCollection routes) {
routes.MapRoute(
"EmployeeByJoinDate",
"Employee/Join/{year}/{month}",
new { controller = "Employee", action = "EmployeeByJoinDate"},
new { year = @"d{4}", month = @"d{2}"}
);
}
Attribute Routes: Advanced Routing in MVC
[Route("Employee/Join/{year:regex(\d{4})}/{month:regex(\d{2})}")]
public ActionResult EmployeeByJoinDate(int year, int month)
{
}
List of in-line Route Constraints
Constraint | Description | Example |
---|---|---|
alpha | Matches uppercase or lowercase Latin alphabet characters (a-z, A-Z) | {x:alpha} |
bool | Matches a Boolean value. | {x:bool} |
datetime | Matches a DateTime value. | {x:datetime} |
decimal | Matches a decimal value. | {x:decimal} |
double | Matches a 64-bit floating-point value. | {x:double} |
float | Matches a 32-bit floating-point value. | {x:float} |
guid | Matches a GUID value. | {x:guid} |
int | Matches a 32-bit integer value. | {x:int} |
length | Matches a string with the specified length or within a specified range of lengths. | {x:length(6)} {x:length(1,20)} |
long | Matches a 64-bit integer value. | {x:long} |
max | Matches an integer with a maximum value. | {x:max(10)} |
maxlength | Matches a string with a maximum length. | {x:maxlength(10)} |
min | Matches an integer with a minimum value. | {x:min(10)} |
minlength | Matches a string with a minimum length. | {x:minlength(10)} |
range | Matches an integer within a range of values. | {x:range(10,50)} |
regex | Matches a regular expression. | {x:regex(^d{3}-d{3}-d{4}$)} |
Route Prefixes:
You can set a common prefix for an entire controller by using the [RoutePrefix] attribute.
Use a tilde (~) on the method attribute to override the route prefix.
[RoutePrefix("Employee/Details")]
public class EmployeeController : Controller
{
// GET Employee/Details
[Route("")]
public IEnumerable EmployeeDetails() {
//...
}
//Employee/Details/12
[Route("{id:int}")]
public EmployeeModels EmployeeDetailsByID(int id) {
//...
}
//employeemodels/Employee/Join/2018/02
[Route("~/Employee/Join/{year:regex(\d{4})}/{month:regex(\d{2})}")]
public HttpResponseMessage EmployeeByJoinDate(int year, int month) {
//...
}
}
Warm UP- Advanced Routing in MVC
- Configure the multiple routes using Map Route extension method in RouteConfig.cs.
- Pass multiple parameter to action by using Route Configuring or Attribute Routes.
- Restrict the value of parameter using Route Constraints on Route Configuring or Attribute Routes.
- You can set a common prefix for an entire controller by using the [RoutePrefix] attribute.
- Use a tilde (~) on the method attribute to override the route prefix.