A dictionary in Python is an unordered collection that stores key-value pairs that map immutable keys to values, just as a conventional dictionary maps words to a definition.
Keys of the dictionary must be unique and of immutable data type such as string, integer and truple but key-value can be repeated and be of any type.
Please read the previous topic for more understanding about Python
List in Python
Tuple in Python
Sets in Python
#Empty Dictionary
Dict = {}
print(Dict)
Dict = dict()
print(Dict)
# With integer Keys
Dict = {1: "Hello", 2 : "World"}
print(Dict)
# With mix Keys
Dict = {1: "Hello", "Name" : "Lipsa"}
print(Dict)
O/P –
{} {} {1: 'Hello', 2: 'World'} {1: 'Hello', 'Name': 'Lipsa'}
Contents
Create a dictionary from a list of tuple
We can also create a dictionary from a list of tuples. Let’s see the below example.
Dict = dict([(1,'India'), (2, 'USA'), (3, 'UK')])
print(Dict)
print(type(Dict))
O/P –
{1: 'India', 2: 'USA', 3: 'UK'} <class 'dict'>
Note:
Diction in python always mappings of unique keys to values.
Dict= {1 : 'India', 2 : 'USA', 1 : 'UK'}
print(Dict)
print(Dict[1])
O/P –
{1: 'UK', 2: 'USA'} UK
Nested Dictionary
Dictionary can able to hold another dictionary inside value.
Dict = {1: 'Lipsa', 2: { 1: 'Hello', 2: 'World'}}
print(Dict)
O/P –
{1: 'Lipsa', 2: {1: 'Hello', 2: 'World'}}
Accessing Elements of Dictionary in Python
Access the items of dictionary refer to its key name. Key can be used inside [] Square brackets or get() method will also help in accessing the element from a dictionary.
The nested dictionary is an unordered collection of dictionaries. So we can not apply index and slicing to access the element in a dictionary in python.
Dict = {1: "Hello", 2 : "World"}
print(Dict)
#Access element in Dictionary
print(Dict[2])
O/P –
{1: 'Hello', 2: 'World'} World
Dict = {"Name":"Rio", "Company":"Microsoft"}
print(Dict)
#Access dictionary element from dictionary by using string key
print(Dict["Name"])
O/P –
{'Name': 'Rio', 'Company': 'Microsoft'} Rio
print(Dict["Name"]+" is working in "+ Dict["Company"])
O/P –
Rio is working in Microsoft
Try to index and slicing on the dictionary in python
Dict = {"Name":"Elizabeth", "Company":"Google"}
# Indexing
print(Dict[1])
O/P –

#Sciling
print(Dict[0:1])
O/P –
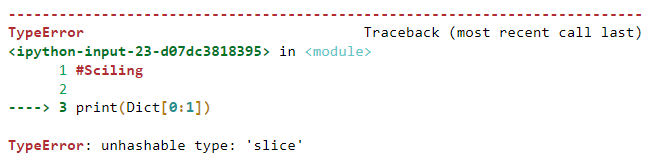
Note:
Indexing and Sciling are not supported in dictionary in python.
No existing key in dictionary If a key does not exist in a dictionary and try to retrieve the data then we will get an error.
Let’s see the below example.
print(Dict["Address"])
O/P –

Access element from Nested Dictionary
We are using the below code to access the element from a nested dictionary in python.
Syntax to access value from a nested dictionary,
Dict = { ‘Key1’: {‘NestKey’: {‘SubNestKey’: ‘Value’}}}
Dict[‘key1’][‘NestKey’][‘SubNestKey’]
Dict = {1: 'Lipsa', 2: { 1: 'Hello', 2: 'World'}}
print(Dict)
print(Dict[2][2])
O/P –
{1: 'Lipsa', 2: {1: 'Hello', 2: 'World'}} 'World'
Access the element by using get method
You can prevent this error by using the dictionary method get, Which normally returns it’s arguments corresponding value. If that is not found, get returns none.
Dict = {"Name":"Elizabeth", "Company":"Google"}
print(Dict.get("Name"))
O/P –
Elizabeth
Note:
If a key does not exist in a dictionary then not get any error but it returns None.
print(Dict.get("Address"))
O/P –
None
Updating and Adding Dictionary elements
Directly update a value in the dictionary by its key. You can update a key’s associated value in an assignment.
Dict = {"Name":"Elizabeth", "Company":"Google"}
Dict["Name"] = "Lipsa"
print(Dict)
O/P –
{'Name': 'Lipsa', 'Company': 'Google'}
Update value by using update method in Dictionary
Python provides an inbuild function to update the data in the dictionary.
# We can write the below code to update value in Dictionary
Dict.update(Name = "Ethan")
print(Dict)
O/P –
{'Name': 'Ethan', 'Company': 'Google'}
Note:
You can insert key-value pairs in the existing dictionary by using the above technique.
Dict = {"Name":"Elizabeth", "Company":"Google"}
Dict["Country"] = "USA"
print(Dict)
O/P –
{'Name': 'Elizabeth', 'Company': 'Google', 'Country': 'USA'}
#Add the element by using update method
Dict.update({"Designation" : "Developer"})
print(Dict)
O/P –
{'Name': 'Elizabeth', 'Company': 'Google', 'Country': 'USA', 'Designation': 'Developer'}
Note:
The update() method adds element(s) to the dictionary if the key is not in the dictionary. If the key is in the dictionary, it updates the key with the new value.
Delete in Dictionary
- del Deletion of keys can be done by using the del keywords and item in nested dictionary can also be deleted by using del keywords.
- pop()/popitem() These can also be used for deleting specific value and arbitary value from a dictionary.
- Clear() All items from a dictionary can be deleted at once by using clear().
D = {'Name': 'Elizabeth', 'Company': 'Google', 'Country': 'USA', 'Designation': 'Developer'}
print(D)
O/P –
{'Name': 'Elizabeth', 'Company': 'Google', 'Country': 'USA', 'Designation': 'Developer'}
del Keyword
del Deletion of keys can be done by using the del keywords and items in the nested dictionary in python can also be deleted by using del keywords.
#Delect element by using del keyword
del D["Country"]
print(D)
O/P –
{'Name': 'Elizabeth', 'Company': 'Google', 'Designation': 'Developer'}
#If key is not exist in Dictionary then it raised the error.
del D["PhoneNo"]
O/P –

#Delete entirely dictionary in python.
del D
print(D)
O/P –

The above code has raised the error because the dictionary is not available.
pop()/popitem()
These can also be used for deleting specific values and arbitrary values from a dictionary in python.
Let’s see the below example.
D = {'Name': 'Elizabeth', 'Company': 'Google', 'Country': 'USA', 'Designation': 'Developer'}
#Return deleting element's value
D.pop("Country")
O/P –
'USA'
print(D)
O/P –
{'Name': 'Elizabeth', 'Company': 'Google', 'Designation': 'Developer'}
Note:
If a key does not exist in the dictionary and we are applying the pop method then it will raise the error.
# Respective key is not exist
D.pop("Address")
O/P –
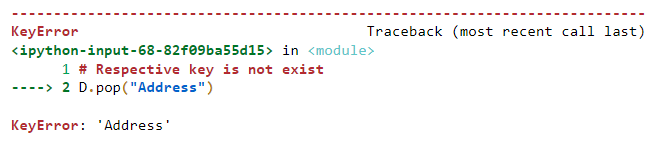
Note:
Popoitem method will not take any parameter.
D = {'Name': 'Elizabeth', 'Company': 'Google', 'Country': 'USA', 'Designation': 'Developer'}
D.popitem()
O/P –
('Designation', 'Developer')
print(D)
O/P –
{'Name': 'Elizabeth', 'Company': 'Google', 'Country': 'USA'}
D.popitem("Country")
O/P –

Clear()
Dict = {1: "Hello", 2 : "World"}
print(Dict)
Dict.clear()
#After Clear
print(Dict)
O/P –
{1: 'Hello', 2: 'World'} {}
Dictionary Membership
We can check if a key is in a dictionary or not using the keyword in. Notice that the membership test is only for the keys and not for the values.
Dict = {1: "Hello", 4 : "World"}
print(1 in Dict)
print(4 not in Dict)
O/P –
True False
Iterating Through a Dictionary in Python
We can iterate through each key in a dictionary using a for loop.
EmpDetails = {'Name': 'Elizabeth', 'Company': 'Google', 'Country': 'USA', 'Designation': 'Developer'}
for i in EmpDetails:
print(EmpDetails[i])
O/P –
Elizabeth Google USA Developer
The above iteration uses the index base. Now we will see some built-in functions for iteration.
item() method is used to return each Key-Value pair as a tuple.
EmpDetails = {'Name': 'Elizabeth', 'Company': 'Google', 'Country': 'USA', 'Designation': 'Developer'}
for k, v in EmpDetails.items():
print(f'{k} : {v}')
O/P –
Name : Elizabeth Company : Google Country : USA Designation : Developer
Similarly, Keys and values can be used to iterate only a dictionary’s key or value respectively.
for k in EmpDetails.keys():
print(f'{k}')
O/P –
Name Company Country Designation
for v in EmpDetails.values():
print(f'{v}')
O/P –
Elizabeth Google USA Developer
Dictionary Methods
Copy Method
It returns a shallow copy of the dictionary in python.
D1 = {1: "Hello", 2 : "World"}
D2 = D1.copy()
print(D2)
O/P –
{1: 'Hello', 2: 'World'}
Len() Method
This function is the count of key entities of the dictionary elements.
D1 = {1: "Hello", 2 : "World"}
print(len(D1))
O/P –
2
fromkeys
It returns a new dictionary in python with keys from sequence and value.
Drinks = {}.fromkeys(['Tea', 'Coffee', 'Cappuccino'], 30)
print(Drinks)
O/P –
{'Tea': 30, 'Coffee': 30, 'Cappuccino': 30}